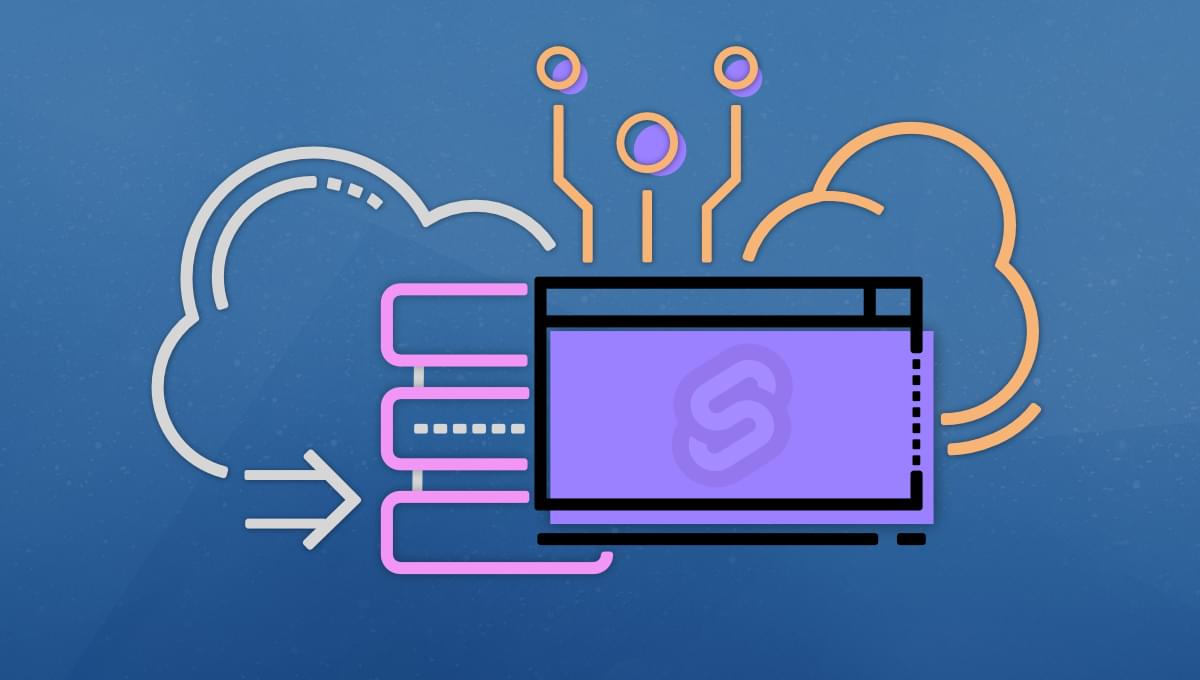
This tutorial explores how you can consume and render data from an API in your Svelte application. You can interact with APIs in Svelte in the onMount()
lifecycle hook using either Axios, Apisauce, JavaScript’s native Fetch API, or any HTTP client of your choice.
We’ll build a sample application that interacts and displays data provided by a REST API server. This application will allow users to fetch lists of blog posts from a REST API and display them on the page.
Prerequisites
In order to follow this tutorial, you’ll need to have some prior knowledge of JavaScript and CSS, as well as some familiarity with Svelte.
You’ll also need Node and npm installed on your machine, as well as Git.
What Is a REST API?
The acronym API stands for “application programming interface”, and in simple terms, it’s a way for two applications to communicate — or share data with each other.
A REST API is a type of API that implements the representational state transfer (REST) protocol. REST is an architectural style for building web services that interact via an HTTP protocol. The request structure of REST includes four essential parts, which are the HTTP method, an endpoint, headers, and the request body.
HTTP Methods
The HTTP method in an API request tells the server what kind of action the client expects it to perform. The most widely used HTTP methods today include GET, POST, PATCH, DELETE and are explained briefly below.
GET
: used to fetch or read information from a serverPOST
: used to create or store records in a serverPUT
/PATCH
: used to update or patch records in a serverDELETE
: used to delete one or more records from a resource point
HTTP Endpoints
An HTTP endpoint in basic terms is an address or URL that specifies where one or more resources can be accessed by an API.
HTTP Headers
HTTP headers are key-value pairs that let the client pass information to the server in a request and vice-versa in a response.
Request Body
The body of an API call is the payload (or data) sent from the client to the server.
Setting Up our Svelte App
We’ll build a sample application that interacts with an external REST API to fetch a list of blog posts from a server. We’ll then display this list on the Svelte client.
We’re not going to dive too much into bundling and the infrastructure of Svelte apps in this tutorial, so we’ll follow the instructions on the official Svelte site to get an application up and running.
In your preferred directory, run:
npx degit sveltejs/template svelte-demo-app
Then, enter into the folder, install the required dependencies using npm and start a development server:
cd svelte-demo-app
npm install
npm run dev --open
You should now see a “Hello, World!” message displayed in your browser at http://localhost:5000/.
Using the Fetch API to Consume a REST API
In this article, we’ll examine two methods of fetching data from an API. Firstly, we’ll look at using the Fetch API, which is native to JavaScript. Then in the next section we’ll look at using the Axios client, before briefly comparing and contrasting the two methods after that.
What is the Fetch API?
The Fetch API is a promise-based mechanism that allows you to make asynchronous API requests to endpoints in JavaScript. If you’re familiar with the XMLHttpRequest()
method, you’ll probably agree that the Fetch API is an improvement — in the sense that it provides additional features such as data caching, the ability to read streaming responses, and more.
Using the Fetch API is as easy as calling the fetch()
method with the path to the resource you’re fetching as a required parameter. For example:
const response = fetch('your-api-url.com/endpoint');
Passing more parameters in a request
The fetch()
method also allows you to be more specific with the request you’re making by passing an init
object as an optional second parameter.
The init
object allows you pass extra details along with your request. The most common of these are listed below:
method
: a string that specifies what HTTP method is being sent to the server and can be one of GET, POST, PUT, PATCH or DELETE.cache
: a string that specifies if the request should be cached. Allowed options aredefault
,no-cache
,reload
,force-cache
,only-if-cached
.headers
: an object used to set headers to be passed along with the request example.body
: an object most commonly used inPOST
,PUT
orPATCH
requests. It allows you pass a payload to the server.
Building out the App
component
Once your Svelte scaffolding has been completed, open up the src
folder and locate the App.svelte
component. This is what is rendered when you visit the project’s home page.
As you can see, the component contains a <script>
block for our JavaScript, a <style>
block for our styles, as well as a <main>
tag with our markup. This is the basic anatomy of a Svelte component.
Let’s start by importing the onMount
hook from Svelte, like so:
import { onMount } from "svelte";
The onMount
hook in Svelte is a lifecycle method used to define instructions that should be carried out once the component where it’s used is first rendered in the DOM tree.
If you’re coming from a React background, you should notice that onMount
hook in Svelte works similarly to the componentDidMount()
method in class-based React components or the useEffect()
hook in React functional components.
Next, we’re going to define a variable to hold the URL of the endpoint we intend to use:
const endpoint = "https://jsonplaceholder.typicode.com/posts";
Note: JSONPlaceholder is a handy, free, online REST API that you can use whenever you need some fake data.
Next, create a posts
variable and assign an empty array to it:
let posts = [];
This empty posts
array is going to be filled up with the data we receive from our API once we make the call.
Finally, we can now make use of the onMount()
method to make a GET
request to the endpoint using JavaScript’s Fetch API as shown below:
onMount(async function () {
const response = await fetch(endpoint);
const data = await response.json();
console.log(data);
});
When pieced together, your App
component should contain the following:
<script>
import { onMount } from "svelte";
const endpoint = "https://jsonplaceholder.typicode.com/posts";
let posts = [];
onMount(async function () {
const response = await fetch(endpoint);
const data = await response.json();
console.log(data);
});
export let name;
</script>
<main>
<h1>Hello {name}!</h1>
<p>Visit the <a href="https://svelte.dev/tutorial">Svelte tutorial</a> to learn how to build Svelte apps.</p>
</main>
<style>
/* ommitted for brevity */
</style>
To check this is working, save the file, then visit http://localhost:3000/ and check the browser’s dev tools. You should see an array of objects logged to the console.
Note: if you’re wondering about that export let name;
statement, this is how we define props in Svelte. The export
keyword here declares that this value is a prop that will be provided by the component’s parent.
Continue reading
How to Fetch Data in Svelte
on SitePoint.